Today we're thrilled to announce that we have tagged the Laravel 10.0 release!
Laravel 10 continues the improvements made in Laravel 9 by introducing argument and return types to all application skeleton methods, as well as all stub files used to generate classes throughout the framework. In addition, a new, developer-friendly abstraction layer has been introduced for starting and interacting with external processes. Further, Laravel Pennant has been introduced to provide a wonderful approach to managing your application's "feature flags".
To get all of the juicy details about this release, check out our official release notes and upgrade guide. We'll cover some of the highlights below.
Method Signature + Return Types
On its initial release, Laravel utilized all of the type-hinting features available in PHP at the time. However, many new features have been added to PHP in the subsequent years, including additional primitive type-hints, return types, and union types.
Laravel 10.x thoroughly updates the application skeleton and all stubs utilized by the framework to introduce argument and return types to all method signatures. In addition, extraneous "doc block" type-hint information has been deleted:
<?php
namespace App\Http\Controllers;
use App\Models\Flight;
use Illuminate\Http\RedirectResponse;
use Illuminate\Http\Request;
use Illuminate\Http\Response;
class FlightController extends Controller
{
/**
* Display a listing of the resource.
*/
public function index(): Response
{
//
}
/**
* Display the specified resource.
*/
public function show(Flight $flight): Response
{
//
}
// ...
}
This change is entirely backwards compatible with existing applications. Therefore, existing applications that do not have these type-hints will continue to function normally.
Laravel Pennant
A new first-party package, Laravel Pennant, has been released. Laravel Pennant offers a light-weight, streamlined approach to managing your application's feature flags. Out of the box, Pennant includes an in-memory array
driver and a database
driver for persistent feature storage.
Features can be easily defined via the Feature::define
method:
use Laravel\Pennant\Feature;
use Illuminate\Support\Lottery;
Feature::define('new-onboarding-flow', function () {
return Lottery::odds(1, 10);
});
Once a feature has been defined, you may easily determine if the current user has access to the given feature:
if (Feature::active('new-onboarding-flow')) {
// ...
}
Of course, for convenience, Blade directives are also available:
@feature('new-onboarding-flow')
<div>
<!-- ... -->
</div>
@endfeature
Pennant offers a variety of more advanced features and APIs. For more information, please consult the comprehensive Pennant documentation.
Processes
Laravel 10.x introduces a beautiful abstraction layer for starting and interacting with external processes via a new Process
facade:
use Illuminate\Support\Facades\Process;
$result = Process::run('ls -la');
return $result->output();
Processes may even be started in pools, allowing for the convenient execution and management of concurrent processes:
use Illuminate\Process\Pool;
use Illuminate\Support\Facades\Pool;
[$first, $second, $third] = Process::concurrently(function (Pool $pool) {
$pool->command('cat first.txt');
$pool->command('cat second.txt');
$pool->command('cat third.txt');
});
return $first->output();
In addition, processes may be faked for convenient testing:
Process::fake();
// ...
Process::assertRan('ls -la');
For more information on interacting with processes, please consult the comprehensive process documentation.
Horizon / Telescope Facelift
Horizon and Telescope have been updated with a fresh, modern look including improved typography, spacing, and design:
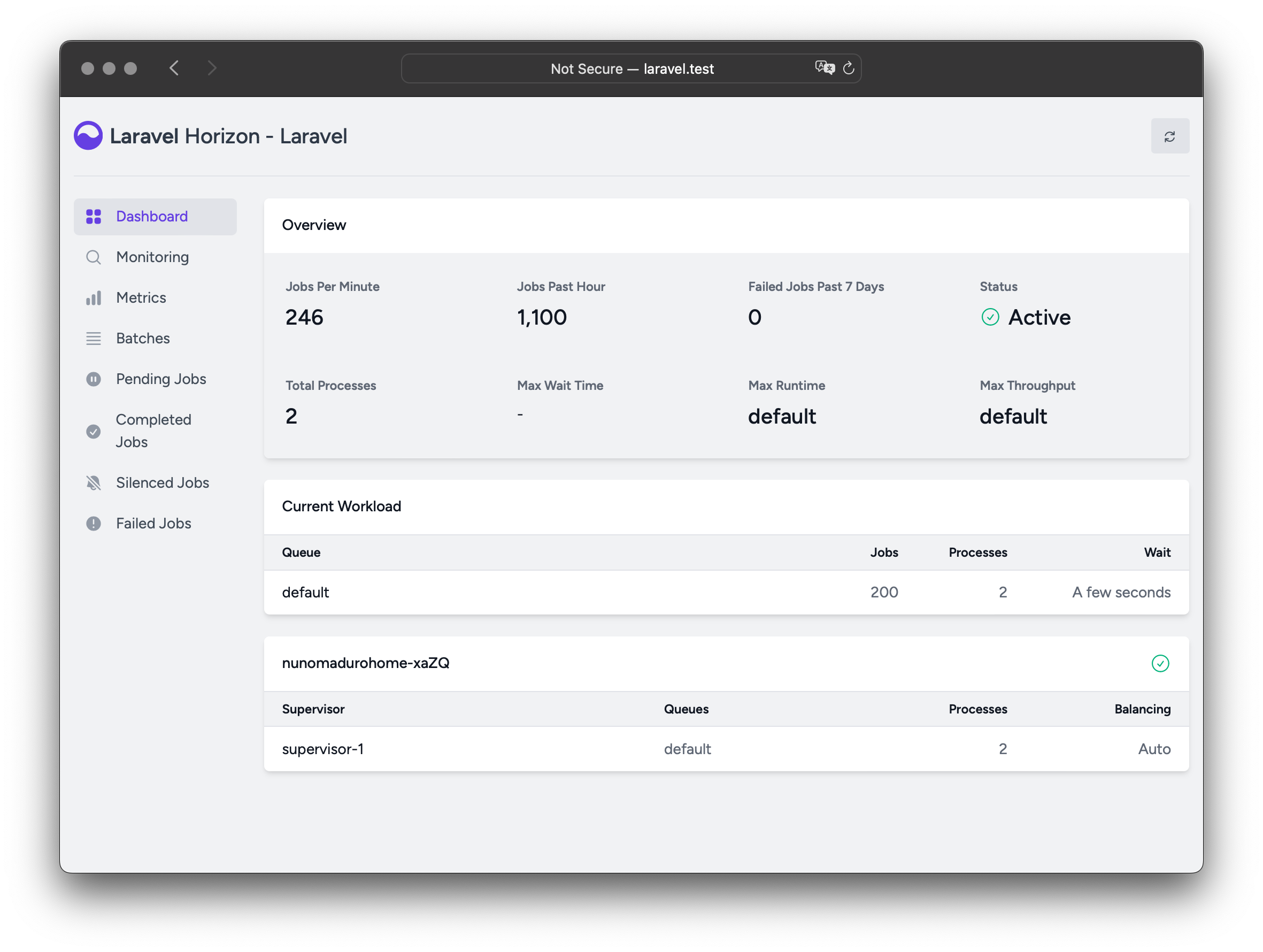